Introduction
Lambda expressions, introduced in Java 8, represent one of the most significant additions to the language. They enable functional programming capabilities in Java, allowing for a more concise, readable, and flexible way of writing code. This blog will dive deep into lambda expressions, exploring their syntax, use cases, benefits, and practical examples to help you master their usage.
What are lambda expressions?
Lambda expression are essentially anonymous methods (or functions) that can be treated as first-class citizens in the language. They allow you to define methods in a more compact form and pass them around as parameters or store them in variables.
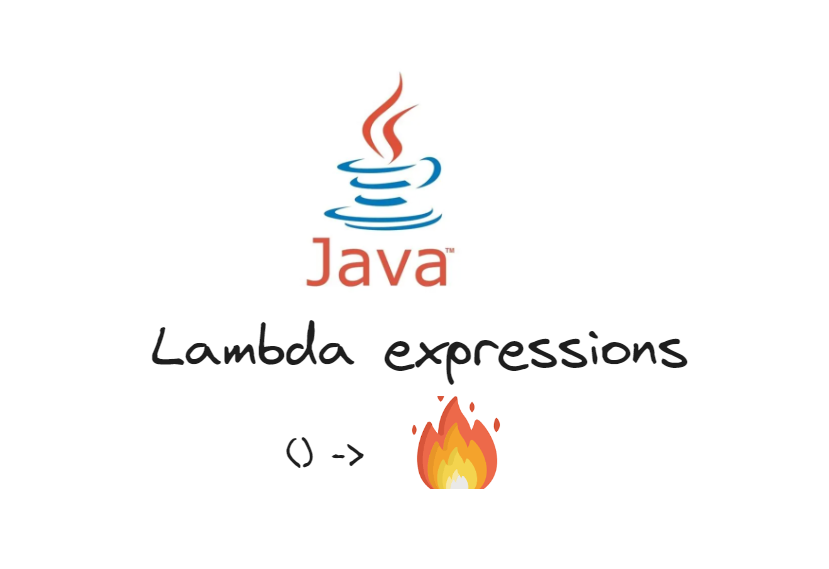
Benefits of lambda expressions
- Conciseness: Reduce boilerplate code and make your code more readable.
- Improved Readability: Simplify code by eliminating the need for anonymous class declarations.
- Functional Programming: Enable functional programming features in Java, such as passing functions as aguments.
- Enhances Collections API: Work seamlessly with the new Stream API introduced in Java 8.
Syntax of lambda expression
The syntax of a lambda expression is straightforward and consists of three parts
- Parameter List: Enclosed in parentheses ()
- Arrow Token: The -> symbol separates the parameters list from the body.
- Body: The code to be executed, which can be either a single expression or a block of code.
Basic Syntax
(parameters) -> expression
(parameters) -> { statements; }
Examples of Lambda Expressions
Runnable r = () -> System.out.println("Hello, World!");
r.run();
Using Lambda Expressions with Functional Interfaces
A functional interface is an interface that contains exactly one abstract method. They can have multiple default or static methods but only one abstract method. Lambda expressions can be used to provide implementations for these abstract methods.
Example Functional Interface
@FunctionalInterface
interface MyFunctionalInterface {
void execute();
}
Using a lambda expression
MyFunctionalInterface myFunc = () -> System.out.println("Executing...");
myFunc.execute();
Common Functional Interfaces in Java
Java provides several built-in functional interfaces in the `java.util.function` package:
`Predicate<T>`: Represents a boolean-valued function of one argurment.
Predicate<String> isEmpty = s -> s.isEmpty();
System.out.println(isEmpty.test("")); // true
`Function<T,R>`: Represents a function that produces a result of type `R` from an input of type `T`
Function<String, Integer> length = s -> s.length();
System.out.println(length.apply("Hello")); // 5
`Consumer<T>`: Represents an operation that accepts a single input argument and returns no result.
Consumer<String> print = s -> System.out.println(s);
print.accept("Hello"); // Hello
`Supplier<T>`: Represents an operation upon two operands of the same type, producing a result of the same type.
BinaryOperator<Integer> multiply = (a, b) -> a * b;
System.out.println(multiply.apply(2, 3)); // 6
Lambda expression with Collections
Lambda expressions are particularly useful when working with collections. They can be used with the enhanced Collections API methods such as `forEach`, `map`. `filter`, `reduce`.
Example: Iterating Over a List
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
Example: Filtering a List
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames); // [Alice]
Example: Mapping a List
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<Integer> lengths = names.stream()
.map(name -> name.length())
.collect(Collectors.toList());
System.out.println(lengths); // [5, 3, 7]
Example: Reducing a list
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, (a, b) -> a + b);
System.out.println(sum); // 15
Advanced Use Cases
@FunctionalInterface
interface MathOperation {
int operate(int a, int b);
}
public class LambdaExample {
public static void main(String[] args) {
MathOperation addition = (a, b) -> a + b;
MathOperation multiplication = (a, b) -> a * b;
System.out.println("Addition: " + addition.operate(5, 3)); // 8
System.out.println("Multiplication: " + multiplication.operate(5, 3)); // 15
}
}
Using Lambdas with Threads
public class LambdaThreadExample {
public static void main(String[] args) {
Runnable task = () -> {
for (int i = 0; i < 5; i++) {
System.out.println("Hello from thread " + i);
}
};
Thread thread = new Thread(task);
thread.start();
}
}
Conclusion
Lambda expressions have brought a significant improvement to Java language by enabling concise, readable, and expressive code. They are a cornerstone of functional programing in Java, making it easier to work with collections, implement functional interfaces, and write cleaner and more maintainable code.
By mastering lambda expressions, you unlock a powerful tool in your Java programing arsenal, allowing you to write more efficient and elegant code. Experiment with different use cases and explore the fill potential of lambda expressions in your Java applications