Spring Security is a powerful and highly customizable authentication and access-control framework for java applications, primarily with Spring Framework. It is an essential tool for developers aiming to secure their applications against various types of attacks and unauthorized access. In this blog post, we’ll dive deep into what Spring Security is, why it’s crucial for modern applications, and how you can get started with it.
Why is Spring Security?
Spring Security is part of the large Spring Framework ecosystem and provides comprehensive security services for java applications. It addressed the common security of enterprise applications, such as authentication, authorization, and protection against common vulnerabilies.
Key Features of Spring Security
- Authentication and Authorization:
- Authentication: Verifies the identity of a user or system. Spring Security supports multiple authentication mechanisms including in-memory, JDBC, LDAP, and OAuth2.
- Authorization: Determines whether a user has the necessary permissions to access a resource. It supports role-based and permission-based access controll.
- Password management:
- Secure password hashing and storage.
- Password encoding using bcrypt, PBKDF2, SCrypt, and other strong hashing algorithms.
- Integration:
- Seamless integration with various authentication provider and protocols.
- Out-of-the-box support for OAuth2 and OpenID Connect.
- Protection Against Attacks:
- CSRF (Cross-Site Request Forgery) protection.
- Protection against session fixation attacks.
- XSS (Cross-Site Scripting) prevention.
- Content Security Policy (CSP) enforcement.
- Method security
- Secure method invocations using annotations like @Secured and @PreAuthorize.
- Fine-grained control over who can invoke specific methods or access particular beans.
Why Use Spring Security
- Robustness: Spring Security offers a comprehensive set of features that cover most of the security needs of a typical enterprise application.
- Customizability: It is highly configurable and can be customized to meet specific requirments.
- Integration: As part of the Spring ecosystem, it integrate seamlessly with other Spring projects like Spring Boot, making it easy to secure applications developed using the Spring framework.
- Community Support: With a large community and extensive documentation, finding support and resources for Spring Security is straightforward.
Getting Started with Spring Security
Here’s a quick example of setting up basic authentication with Spring Security in a Spring Boot application:
Add Spring Security Dependency
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Configure Security
package com.example.spring_basic_auth.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.Customizer;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.crypto.password.NoOpPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.provisioning.InMemoryUserDetailsManager;
import org.springframework.security.web.SecurityFilterChain;
@EnableWebSecurity
@Configuration
public class SecurityConfig {
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.authorizeHttpRequests(requests -> {
requests.anyRequest().authenticated();
})
.httpBasic(Customizer.withDefaults());
return http.build();
}
@Bean
public UserDetailsService userDetailsService() {
UserDetails userDetails = User.builder()
.username("user")
.password("123")
.build();
UserDetailsService userDetailsService = new InMemoryUserDetailsManager(userDetails);
return userDetailsService;
}
@Bean
public PasswordEncoder passwordEncoder() {
return NoOpPasswordEncoder.getInstance();
}
}
Run your application
With the configuration in place, start your Spring Boot application. You should new have a basic authentication setup where the user must provide a username and password to access any endpoint.
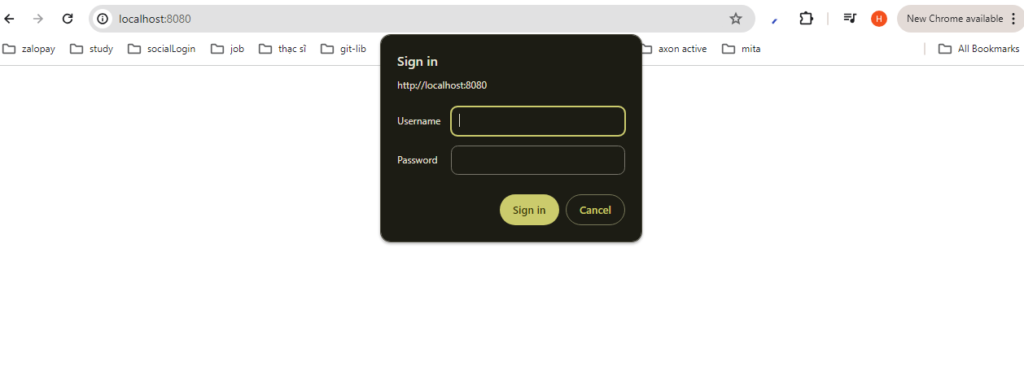
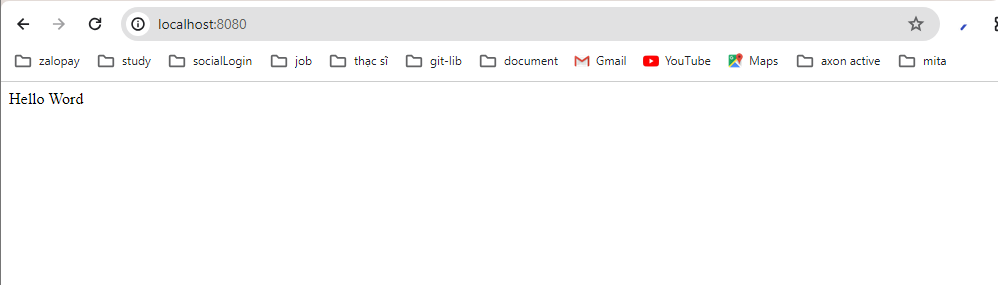
Source Code: https://github.com/hongquan080799/spring-security-example/tree/blog_1_overview
Conclusion
Spring Security is a vital component for securing modern Java applications. It offers a wide range of features and an extensive set of tools for developers to implement robust security measures. Whether you are building a small application or a large enterprise system, Spring Security can provide the necessary mechanisms to protect your application from various security threats.