Introduction
Generics in Java provide a way to define classes, interfaces, and methods with a placeholder for types. allowing for type-safe data structures and algorithms. By using generics, developers can create reusable code components that work with any data type while ensuring type safety at compile-time. This blog will delve into the fundamentals of generics in Java, demonstrate how to define generic classes and methods, and highlight their benefits in creating type-safe collections and methods.
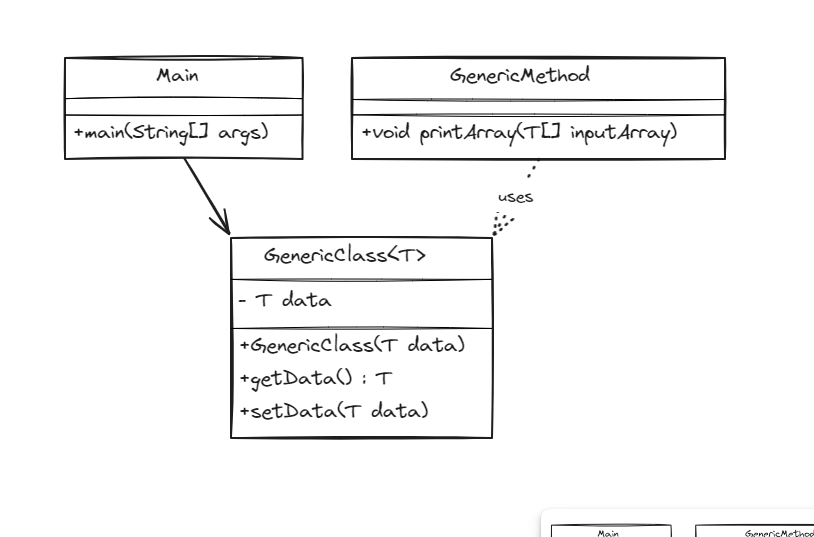
What Are Generics?
Generics were introduced in Java 5 as a means to ensure stronger type checks of compile-time. They allow you to define classed, interfaces, and methods with type parameters, which can be replaced with actual types when the code is executed. This mean you can write more flexible an reusable code while avoiding the common pitfalls of type casting and type mismatches.
Benefits of Generics
- Type Safety: Generics provide compile-time type checking, which reduces runtime errors by ensuring that only compatible types are used.
- Reusability: Generic code can be reusable for different types without modification.
- Elimination of type Casting: Generics eliminate the need for explicit type casting, making code cleaner and easier to read.
- Enhance Performance: By avoiding unnecessary type casting, generics can potentially improve performance.
Defining Generic Classes
A generic class is defined with a type parameter inside angle brackets (`<>`). This type parameter can be used within the class to define fields, methods, and constructors
public class Box<T> {
private T content;
public Box(T content) {
this.content = content;
}
public T getContent() {
return content;
}
public void setContent(T content) {
this.content = content;
}
public static void main(String[] args) {
Box<Integer> integerBox = new Box<>(123);
Box<String> stringBox = new Box<>("Hello Generics");
System.out.println("Integer Box Content: " + integerBox.getContent());
System.out.println("String Box Content: " + stringBox.getContent());
}
}
In this example, `T` is a type parameter that can be replaced with any type. When we create instances of Box, we specify the type (Integer or String), ensuring that only objects of that types can be stored in the box.
Defining Generic Methods
Generic methods allow you to define methods with type parameters. These type parameters can be used in the method signature and body.
public class ArrayUtils {
public static <T> void swap(T[] array, int index1, int index2) {
T temp = array[index1];
array[index1] = array[index2];
array[index2] = temp;
}
public static void main(String[] args) {
Integer[] intArray = {1, 2, 3, 4, 5};
String[] strArray = {"A", "B", "C", "D", "E"};
System.out.println("Before swap: " + Arrays.toString(intArray));
swap(intArray, 0, 4);
System.out.println("After swap: " + Arrays.toString(intArray));
System.out.println("Before swap: " + Arrays.toString(strArray));
swap(strArray, 1, 3);
System.out.println("After swap: " + Arrays.toString(strArray));
}
}
In this example, the swap method is defined with a type parameter T. This allows the method to work with arrays of any type, ensuring type safety while providing reusable functionality.
Bounded Type Parameters
Generics also support bounded type parameters, allowing you to restrict the types that can be used as arguments. This is useful when you need to ensure that that type parameter adheres to a specific interface or superclass.
import java.util.List;
public class NumberUtils {
public static <T extends Number> double sum(List<T> numbers) {
double total = 0.0;
for (T number : numbers) {
total += number.doubleValue();
}
return total;
}
public static void main(String[] args) {
List<Integer> intList = List.of(1, 2, 3, 4, 5);
List<Double> doubleList = List.of(1.1, 2.2, 3.3, 4.4, 5.5);
System.out.println("Sum of integers: " + sum(intList));
System.out.println("Sum of doubles: " + sum(doubleList));
}
}
Conclusion
Generics are a powerful feature in java that enhances type safety, reusability, and readability of your code. By using generics, you can create flexible and reusable classes and methods that work with any type while ensuring that only compatible types are used. Understanding and utilizing generics is essential for writing robust and maintainable Java applications. Whether you’re working with collections, algorithms, or any other type of data structure, generics provide the tools you need to write clean and efficient code.