Introduction
Transaction management is a critical aspect of any enterprise application that interacts with a database. It ensures data consistency and integrity by allowing multiple operations to be treated as a single unit of work. In this blog, we will explore how transaction management is handled in Spring Data JPA, the role of Spring’s @Transactional annotation, and advanced concepts like propagation and isolation levels.
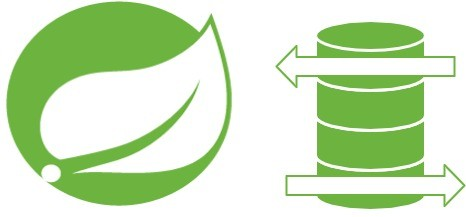
Understanding Transactions in JPA
A transaction is a sequence of operations performed as a single logical unit of work. In JPA, a transaction ensures that either all operations within the transaction are completed successfully, or none are. This all-or-nothing approach guarantees the consistency of your data.
ACID Properties
- Atomicity: Ensures that all operations within a transaction are treated as a single unit. If any operation fails, the transaction is aborted, and all changes made are rolled back.
- Consistency: Ensures that a transaction brings the database from one valid state to another, maintaining database invariants
- Isolation: Ensures that transactions are isolated from each other. One transaction’s intermediate results are invisible to other transactions
- Durability: Once a transaction is committed, its changes are permanent and survive any subsequent failures
In JPA, transactions are typically controlled by the EntityManager. You can begin, commit, or roll back transactions using methods provided by the EntityManager interface. However, managing transactions manually can be cumbersome, which is where Spring’s transaction management feature come in to play.
Spring’s @Transactional Annotation
Spring simplifies transaction management with the @Transational annotation, which can be applied to methods or classes. When a method or class is annotated with @Transactional, Spring manages the transaction boundaries for you beginning the transaction at the start of the method and committing it at the end.
Example of @Transactional
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional
public void createUser(User user) {
userRepository.save(user);
// Other operations can be included, and all will be part of the same transaction
}
}
In this example, if any exception occurs within the createUser method, the entire transaction will be rolled back, ensuring that no partial changes are committed to the database.
Key Features of @Transactional
- propagation: Determines how transactions are propagated when multiple transactional methods are invoked.
- isolation: Defines the isolation level for a transaction, controlling the visibility of changes made by one transaction to others.
- timeout: Specifies the maximum time in seconds that a transaction can run before being rolled back.
- readOnly: Indicates whether the transaction is read-only, allowing for optimization by the underlying database.
- rollbackFor: Specifies which exceptions should trigger a rollback.
- noRollbackFor: Specifies exceptions that should not trigger a rollback.
Propagation and Isolation Levels
Spring’s transaction management allows you to control how transactions behave through propagation and isolation levels.
Propagation Levels
Propagation levels define how transactions are managed when multiple transactional methods are called in succession.
- REQUIRED (default): Joins the existing transaction or creates a new one if none exists.
- REQUIRES_NEW: Suspends the current transaction (if any) and creates a new one.
- NESTED: Executes within a nested transaction if a current transaction exists.
- MANDATORY: Requires an existing transaction; throws an exception if no transaction exists.
- SUPPORTS: Executes non-transactionally if there’s no existing transaction.
- NOT_SUPPORTED: Suspends the current transaction (if any) and executes non-transactionally
- NEVER: Throws an exception if a transaction exists
@Transactional(propagation = Propagation.REQUIRES_NEW)
public void updateUser(User user) {
userRepository.save(user);
// This method will always run in a new transaction
}
Isolation Levels
Isolation levels define how the data affected by a transaction is visible to other concurrent transactions
- READ_UNCOMMITED: The lowest isolation level; allows dirty reads.
- READ_COMMITED: Prevents dirty reads; a transaction can only read committed data.
- REPEATABLE_READ: Prevents dirty and non-repeatable reads
- SERIALIZABLE: The highest isolation level; completely isolates the transactions from each other.
@Transactional(isolation = Isolation.SERIALIZABLE)
public void transferFunds(Account from, Account to, BigDecimal amount) {
// Ensures complete isolation of this transaction
from.debit(amount);
to.credit(amount);
accountRepository.save(from);
accountRepository.save(to);
}
Managing Transactions Across Multiple Data Sources
In complex applications, you may need to manage transactions across multiple databases or data sources. Spring provides support for this through JTA (Java Transaction API) and XA transactions.
When working with multiple data sources, you typically define multiple DataSource beans in your Spring configuration. You can the use JTA to manage distributed transactions that span across these multiple data sources
@Configuration
public class DataSourceConfig {
@Bean
public DataSource dataSource1() {
// Configure and return the first DataSource
}
@Bean
public DataSource dataSource2() {
// Configure and return the second DataSource
}
@Bean
public PlatformTransactionManager transactionManager() {
return new JtaTransactionManager();
}
}
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.transaction.jta.JtaTransactionManager;
@Service
public class MultiDataSourceService {
@Autowired
private DataSource1Repository repository1;
@Autowired
private DataSource2Repository repository2;
@Transactional(transactionManager = "jtaTransactionManager")
public void performDistributedTransaction() {
repository1.save(new Entity1());
repository2.save(new Entity2());
// Operations on both data sources are part of the same transaction
}
}
In this example, the performDistributedTransaction method will execute operations on both data sources as part of a single, distributed transaction. If any part of the transaction fails, all changes will be rolled back across all involved data sources.
Conclusion
Transaction management is essential for maintaining data consistency and integrity in your applications. Spring Data JPA, combined with the power of Spring’s @Transactional annotation, provides a robust and flexible way to manage transactions. Whether dealing with simple transactions or complex distributed transactions across multiple data sources, Spring Data JPA allows you to handle efficiently with minimal boilerplate code. Understanding transaction propagation and isolation levels further enhances your ability to control transaction behavior in various scenarios.