Given an integer x, return true if x is a palindrome, and false otherwise.
Example 1:
Input: x = 121 Output: true Explanation: 121 reads as 121 from left to right and from right to left.
Example 2:
Input: x = -121 Output: false Explanation: From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
Example 3:
Input: x = 10 Output: false Explanation: Reads 01 from right to left. Therefore it is not a palindrome.
Solution Approach
To determine if a number is a palindrome, we can reverse its digits and compare it to the original number. If they are equal, the number is a palindrome. Here, I’ll show you two solutions for achieving this:
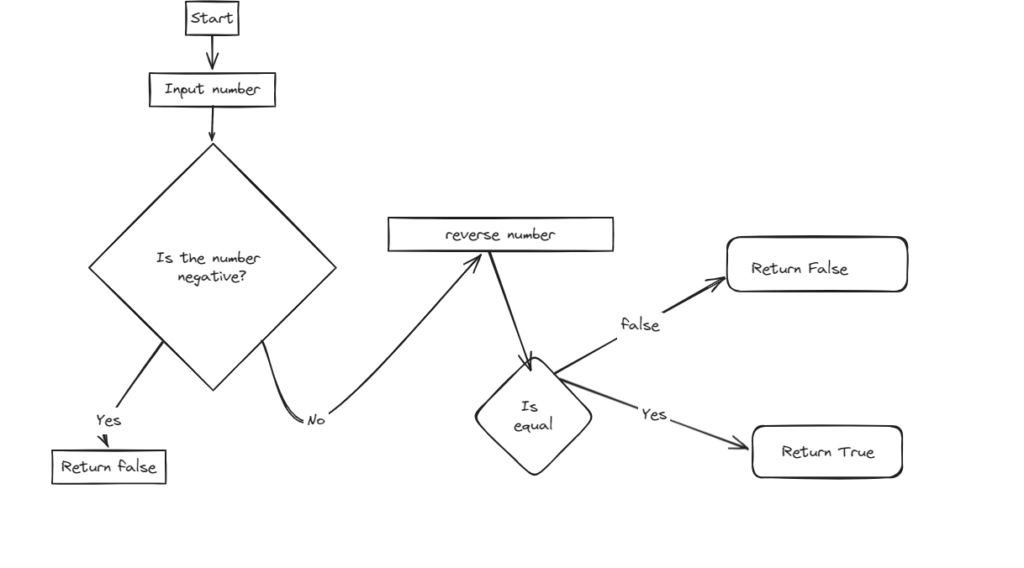
Solution 1: Reverse the entire number
In order to reverse the entire number, we extract the last digit using the modulo operator (%) and add it to the reversed number: reversedNum = reversedNum * 10 + number % 10. We then divide number by 10 to remove the last digit. This loop continues until the original number becomes less than or equal to 0.
class Solution:
def isPalindrome(self, x: int) -> bool:
number = x
reversedNumber = 0;
while number > 0:
reversedNumber = reversedNumber * 10 + number % 10
number = number // 10
return True if reversedNumber == x else False;
Solution 2: Reverse the half number
We can actually reverse just half of the number and still determine if it’s a palindrome. For example
- 123456654321: 654321 -> 123456 then compare the half first, we can see they are equal so return True
- 12345654321: 654321 -> 123456 then compare the half first, se can see they are equal in this comparation: 123456 / 10 == 12345
class Solution:
def isPalindrome(self, x: int) -> bool:
number = x
reversedNumber = 0;
while number > reversedNumber:
reversedNumber = reversedNumber * 10 + number % 10
number = number // 10
return True if reversedNumber == number or reversedNumber == number / 10 else False;
Source Code: https://github.com/hongquan080799/leetcode/tree/master/palidrome_number