Introduction of ORM
Object-Relational Mapping (ORM) is a technique that allows developers to interact with a database using an object-oriented paradigm. This abstraction simplifies databases operations by allowing developers to work with java objects instead of SQL statements. ORM tools automatically handle conversion between database tables and java objects, making it easier to manage data persistence.
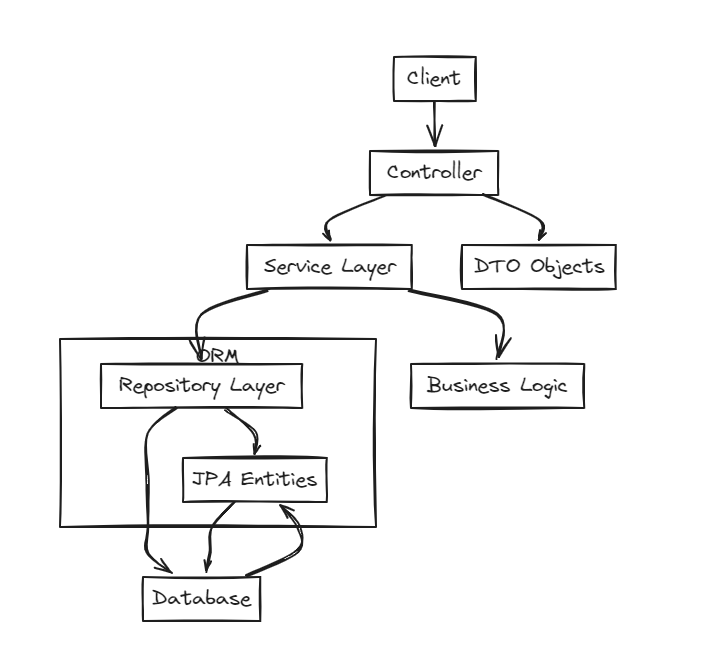
Early Days of ORM
Before ORM tools, developers manually wrote SQL queries to interact with databases. This approach was error-prone and hard to maintain, especially as applications grew in complexity. The need for a more intuitive way to handle database operations led developers to the development of ORM frameworks.
Hibernate: The Pioneer
One of the most influential ORM frameworks in the java ecosystem is Hibernate. Released in early 2000s, Hibernate provided a robust solution for ORM by offering features such as:
- Transparent persistence
- Object-oriented query language (HQL)
- Lazy loading
- Caching
Hibernate quickly became popular due to its flexibility and ease of use, setting the standard for ORM in java applications.
Spring Framework and Hibernate
The Spring Framework, introduced in 2003, aimed to simplify java enterprise development by promotion best practices such as dependency injection and aspect-oriented programming. Given Hibernate’s popularity, Spring integrated with Hibernate to provide a seamless ORM experience. The Spring ORM module allowed developers to use Hibernate in a Spring application, benefiting from Spring’s dependency injection and transaction management capabilities.
JPA: Standardizing ORM
In 2006, the java Persistence API(JPA) was introduced as part of java EE 5. JPA provided a standardized API for ORM, enabling developers to switch between different ORM implementations without changing their code. Hibernate implemented the JPA specification, further solidifying its position as the leading ORM framework.
SpringBoot: Simplifying Application Development
Spring Boot, introduced in 2014, revolutionized Spring application development by providing a convention-over-configuration approach. It aimed to simplify the setup and configuration of Spring applications, allowing developers to get started quickly without extensive XML configuration.
Spring Boot integrate seamlessly with JPA and Hibernate, offering auto-configuration and sensible defaults. This integration made it easier than ever to set up and use ORM in Spring applications. Developers could simply include the Spring Boot Starter Data JPA dependency in their project, and Spring Boot would automatically configure the necessary components.
Key Features of Spring Boot ORM
- Auto-Configuration: Spring Boot automatically configures Hibernate and JPA based on the project’s dependencies and properties.
- Stater POMs: Spring Boot provides starter POMs like `spring-boot-starter-data-jpa`, which include all necessary dependencies for using JPA and Hibernate.
- Embedded Databases: For development and testing, Spring Boot supports embedded databases like H3, HSQLDB, and Derby, making it easy to get started without an external database.
- Database Migrations: Integration with tools like Flyway and Liquibase simplifies database versioning and migrations.
- Spring Data JPA: This Spring module provides a higher-level abstraction over JPA. reducing boilerplate code by offering repository interfaces for common CURD operations.
Comparation between JPA, Hibernate, Spring Data JPA
JPA (Java Persistence API):
- JPA is a specification, a set of standards and interfaces defined by Oracle that outlines how to perform object-relational mapping (ORM) in Java applications.
- It provides a way to transparently persist Java objects to a relational databases and vice versa.
- JPA doesn’t offer a concrete implementation – it defines what needs to be done, now how.
Hibernate
- Hibernate is a powerful open-source implementation of the JPA specification.
- It provides a mature and feature-rich framework for object-relational mapping.
- Hibernate translates between java objects and databases tables, handling persistence details like SQL generation and database interations.
- Since it adheres to the JPA standards, you can use other JPA providers (if needed) with minimal code changes.
Spring Data JPA
- Spring Data JPA builds upon the foundation of JPA by providing a higher-level abstraction for data access in Spring applications.
- Spring Data JPA introduces the concept of repository interfaces, which provides basic CURD operations, auto implementation for your repository interface at runtime
- Support additional features: Pagination, sorting, support for various data stores beyond relational databases (NOSQL)
Conclusion
The journey of ORM in Spring ecosystem reflects the broader evolution of java enterprise development. From the early days of manual SQL to the sophisticated ORM framework of today. All of these make it an indispensable tool for modern Java developer