Introduction to Caching in JPA
Caching is a powerful technique to improve the performance of applications by improve the performance of applications by reducing the number of databases queries. In the context of JPA, caching can significantly speed up the retrieval of entities, particularly when the same data is accessed multiple times within a session or across multiple sessions. Caching in JPA can be broadly categorized into two levels: The first-level cache and the second-level cache.
- First-Level Cache: Also know as the Persistence Context. this cache is associated with the EntityManger and is active throughout the lifecycle of the EntityManger
- Second-Level Cache: This cache is sharing across EntityManager instances and can be configurated to store data beyond lifecycle of a single EntityManager, making it available across sessions.
Understanding and effectively implementing caching in Spring Data JPA can lead to substantial performance gains in your application.
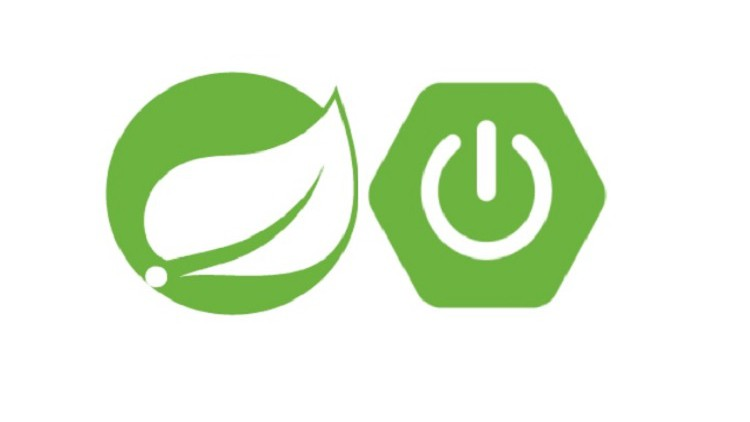
First-Level Cache (Persistence Context)
What is the First-Level Cache?
The first-level cache is intrinsic to the JPA specification and is automatically managed by the JPA provider (Hibernate). It is also known as the Persistence Context. This cache is local to the EntityManager instance, meaning that once an entity is loaded within an EntityManager, it remains in memory for the duration of that instance.
How it works
When you query for an entity, the EntityManager first checks the first-level cache to see if the entity is already loaded. If it is, the entity is returned from the cache instead of querying the database. This minimizes the number of database hits and can significantly boost performance.
The second-level cache is a global cache that is shared among all EntityManager instances in a given application. Unlike the first0level cache, the second-level cache is optional and needs to be explicitly configured. It allows entities to be cached across multiple sessions, making it possible to avoid repeated database queries even across different instances of EntityManager
Configuring the Second-Level Cache
To enable the second-level cache in Spring Data JPA, you need to configure the JPA provider (e.g., Hibernate) and choose a caching provider like EhCache, Hazelcast, or Infinispan.
Example configuration using Ehcache
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-ehcache</artifactId>
<version>5.6.15.Final</version>
</dependency>
In application.properties
spring.jpa.properties.hibernate.cache.use_second_level_cache=true
spring.jpa.properties.hibernate.cache.region.factory_class=org.hibernate.cache.ehcache.EhCacheRegionFactory
spring.jpa.properties.hibernate.cache.use_query_cache=true
Annotate the entity classes to be cached:
@Entity
@Cacheable
@org.hibernate.annotations.Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
public class Product {
@Id
private Long id;
private String name;
private Double price;
// Getters and setters
}
Cache Concurrency Strategies
When configuring the second-level cache, you can choose different concurrency strategies:
- READ_ONLY: Suitable for entities that do not change once persisted
- NONSTRICT_READ_WRITE: Allows for weak consistency; updates may not be visible immediately
- READ_WRITE: Ensures strong consistency, but with a higher performance cost.
- TRANSACTIONAL: Uses JTA to manage transactions
Using @Cachable, @CachePut, and @CacheEvict Annotations
@Cacheable
The @Cacheable annotation is used to indicate that the result of a method or an entity should be cached. When the method is called, Spring first checks the cache. If the result is found, it returns it from the cache; otherwise, it invokes method and stores the result in the cache
@Cacheable(value = "products", key = "#id")
public Product findProductById(Long id) {
return productRepository.findById(id).orElse(null);
}
@CachePut
The @CachePut annotation is used to update the cache with the result of the method, even if the method is not invoked.
@CachePut(value = "products", key = "#product.id")
public Product updateProduct(Product product) {
return productRepository.save(product);
}
@CacheEvict
The @CacheEvict annotation í used to remove entries from the cache. This is useful when the cached data becomes stale, such as after an update or delete operation.
@CacheEvict(value = "products", key = "#id")
public void deleteProduct(Long id) {
productRepository.deleteById(id);
}
Best practices for Caching in JPA
- Choose the Right Cache strategy
- Selecting the appropriate caching strategy (first-level vs second-level) depends on the specific needs of your application. First-level caching is sufficient for most simple use cases, but second-level caching can greatly improve performance in applications with heavy read operations across sessions.
- Cache Only What Is Nescessary
- Caching comes with its own overhead, so it’s important to cache only the data that is frequently accessed and unlikely to change. Over-caching can lead memory issues and stale data problems.
- Use read-Only Caches for Statis Data
- For data that does not change (e.g., reference data like country codes), use a read-only cache. This ensures that the data is served quickly without the risk of inconsistencies
- Invalidate Cache When Data Changes
- Ensures that your cache is invalided or updated when the underlying data changes. Using annotations like @CacheEvict and @CachePut helps manage cache consistency
- Monitor and Tune Cache Performance
- Regularly monitor cache performance and tune configurations as needed. Tools like EhCache’s management console of Spring Boot’s actuator endpoints can be helpful in understanding cache hits/misses and adjusting settings.
- Be Aware of Cache Size
- Carefully configure the cache size to avoid memory exhaustion. Depending on your cache provider, you may be able to set limits on the number of entries, the time-to-live (TTL), or the memory size.
Conclusion
Caching in Spring Data JPA is a powerful tool to optimize the performance of your application. By understanding and effectively utilizing both the first-level and second-level caches, you can reduce database load and improve response times. Annotations like @Cacheable, @CachePut and @CacheEvict provide granular control over caching behavior, allowing best practices, you can ensure that your caching strategy enhances performance without introducing inconsistencies or memory issues.